Currently Empty: £0.00
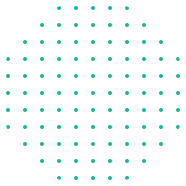
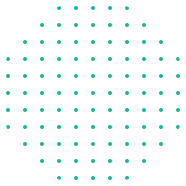
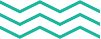
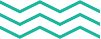
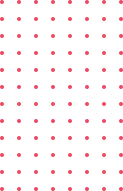
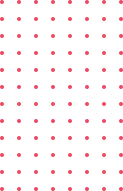
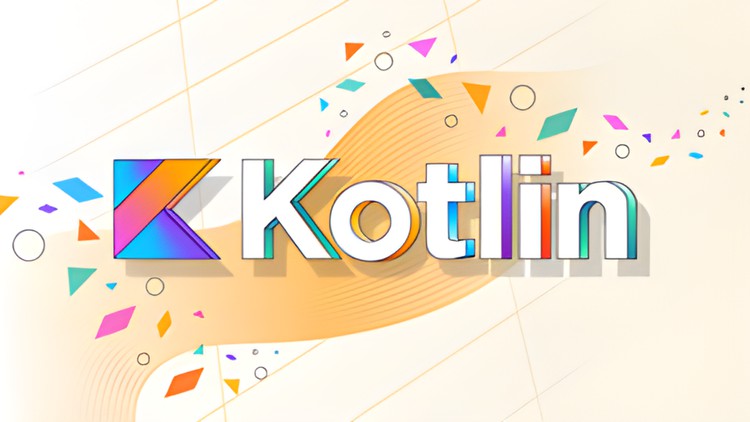
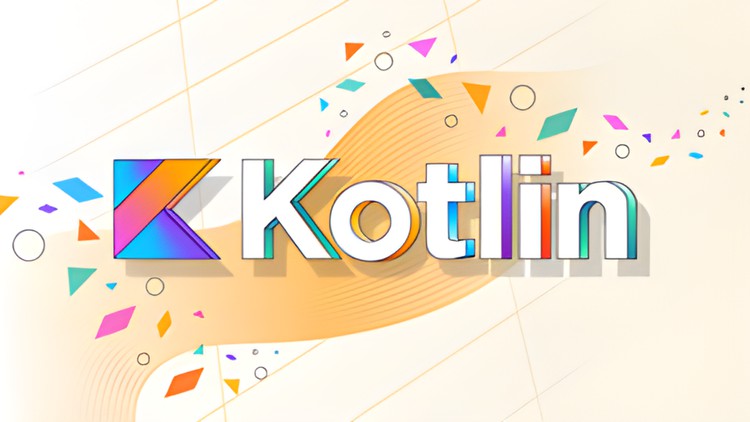
Are you ready to elevate your programming skills and become a Kotlin master with a special focus on Android development? Welcome to “Mastering Kotlin for Android Development in 2024: A Premium Learning Experience”!
In this comprehensive course, we’ll guide you from the fundamentals of Kotlin to advanced mastery, all within the dynamic landscape of 2024. Whether you’re a seasoned developer or just starting your coding journey, this course is meticulously designed to provide you with a top-tier learning experience.
What You’ll Learn:
-
Kotlin Foundations:
-
Build a solid understanding of Kotlin’s syntax, data types, and essential language features.
-
Master the art of writing clean and concise Kotlin code.
-
-
Advanced Kotlin Concepts:
-
Dive deep into advanced Kotlin features, including extension functions, sealed classes, and more.
-
Explore how Kotlin enhances your coding efficiency and expressiveness.
-
-
Object-Oriented and Functional Programming:
-
Understand object-oriented programming principles in Kotlin.
-
Embrace functional programming paradigms for elegant and powerful code.
-
-
Android Development with Kotlin:
-
Learn how to leverage Kotlin for Android app development.
-
Build real-world Android applications with hands-on projects.
-
-
Concurrency and Coroutines:
-
Master Kotlin coroutines for asynchronous and concurrent programming.
-
Enhance your app’s performance and responsiveness with effective concurrency strategies.
-
-
Null Safety and Smart Casting:
-
Eliminate null pointer exceptions with Kotlin’s robust null safety features.
-
Utilize smart casting for safer and more reliable code.
-
-
Delegation and Design Patterns:
-
Explore the power of delegation for code reuse and modular design.
-
Implement common design patterns in Kotlin for scalable applications.
-
-
Unit Testing and Best Practices:
-
Write effective unit tests using JUnit 5 in Kotlin.
-
Adopt best practices for clean code, maintainability, and collaboration.
-
Why Enroll in This Course:
-
Premium Learning Experience:
-
Immerse yourself in a premium course with high-production video content, interactive quizzes, and detailed hands-on exercises.
-
Benefit from a supportive learning environment with personalized assistance from instructors.
-
-
2024 Relevance:
-
Stay ahead in 2024 with content that aligns with the latest trends and advancements in Kotlin and Android development.
-
-
Career Advancement:
-
Boost your career prospects by mastering a sought-after language for Android development.
-
Impress potential employers with your Kotlin proficiency and modern programming skills.
-
Don’t miss out on this opportunity to become a Kotlin expert in 2024! Enroll now and embark on a journey towards mastering Kotlin for Android development in a premium learning environment. Happy coding!
Object-Oriented Programming
-
1Variables
Introduction to Variables:
Understand the concept of variables as containers for storing data in a program.
Explore why variables are crucial for flexible and dynamic programming.
Declaring Variables in Kotlin:
Learn the syntax for declaring variables in Kotlin.
Understand the difference between var (mutable) and val (immutable) variables.
Data Types in Kotlin:
Explore the various data types available in Kotlin, including primitive types like Int, Double, and Boolean.
Understand how to choose the appropriate data type for different kinds of data.
Variable Naming Conventions:
Follow best practices for naming variables in Kotlin to enhance code readability.
Understand the importance of meaningful variable names in writing clean and maintainable code.
Type Inference:
Learn about Kotlin's type inference system, which allows the compiler to automatically determine variable types.
Understand how type inference simplifies variable declarations and enhances code conciseness.
String Interpolation:
Explore the concept of string interpolation in Kotlin.
Learn how to embed variables within strings for dynamic content.
Nullable Types and Null Safety:
Understand the importance of null safety in Kotlin.
Learn how to declare nullable types and handle null values safely.
Constants in Kotlin:
Introduce the concept of constants and how to declare them in Kotlin using the const keyword.
Explore the use of constants for values that remain unchanged throughout the program.
Scope of Variables:
Understand the concept of variable scope in Kotlin.
Learn how the visibility and accessibility of variables are determined by their scope.
Practical Exercises and Examples:
Engage in hands-on exercises and coding examples to reinforce the understanding of variables in Kotlin.
Apply knowledge gained to real-world scenarios and problem-solving.
-
2Data types
Understanding Basic Data Types:
Gain a solid understanding of basic data types in Kotlin, including integers, floating-point numbers, characters, and booleans.
Learn how to declare variables and constants using these fundamental data types.
String Manipulation:
Explore the String data type in Kotlin and learn how to manipulate and concatenate strings.
Understand common string operations, such as substring extraction and length retrieval.
Collections in Kotlin:
Dive into collections like arrays and lists to store and manipulate sets of data.
Learn about the advantages and use cases of different types of collections.
Type Inference:
Understand Kotlin's ability to infer data types, reducing the need for explicit type declarations.
Explore scenarios where type inference enhances code readability and conciseness.
User-Defined Data Types:
Learn how to create user-defined data types using classes and enums in Kotlin.
Understand the principles of object-oriented programming related to data types.
Nullability and Safe Calls:
Grasp the concept of nullability in Kotlin and how it's addressed to prevent null pointer exceptions.
Learn to use safe calls and the ?. operator to safely navigate nullable types.
Smart Casts and Type Checks:
Explore Kotlin's smart cast feature, which allows automatic casting of types in certain contexts.
Understand how to perform type checks using the is operator.
Type Conversion:
Learn about type conversion in Kotlin, both explicitly and implicitly.
Understand how to convert between different data types when necessary.
BigInteger and BigDecimal:
Explore the BigInteger and BigDecimal classes for working with large integers and precise decimal numbers.
Understand use cases and scenarios where these classes are beneficial.
-
3Basic Variable Declaration
-
4Type inference
Implicit Type Declarations:
Understand how Kotlin allows variables to be declared without explicitly specifying their types.
Explore scenarios where the compiler can infer the type based on the assigned value.
Explicit Type Declarations:
Learn when and how to explicitly declare the type of a variable or expression in Kotlin.
Understand situations where explicit type declarations might be beneficial.
Type Inference with Functions:
Explore how type inference works in the context of function return types.
Understand how the compiler deduces the types of function parameters based on usage.
Lambda Expressions and Type Inference:
Learn about type inference when working with lambda expressions.
Understand how Kotlin handles the deduction of parameter types and return types in lambda functions.
Nullable Types and Smart Casts:
Explore how type inference contributes to Kotlin's null safety features.
Learn about smart casts, where the compiler automatically casts types after null checks.
Type Inference with Collections:
Understand how Kotlin infers the types of elements in collections.
Explore concise ways to work with lists, sets, and maps using type inference.
Type Inference in Object Initialization:
Learn how Kotlin infers types during object initialization and construction.
Understand the role of type inference in concise and readable object creation.
Best Practices for Type Inference:
Gain insights into best practices for leveraging type inference effectively.
Understand scenarios where explicit type declarations might be preferred for code clarity.
-
5If, else
Conditional Statements:
Understand the syntax and usage of "if-else" statements in Kotlin.
Learn how to make decisions in code based on conditions.
Expression vs. Statement:
Differentiate between "if" as an expression and "if-else" as a statement in Kotlin.
Explore their use cases and implications in coding scenarios.
Complex Conditions:
Handle more complex scenarios by nesting "if-else" statements.
Gain proficiency in structuring decision-making logic effectively.
-
6Check Number Type
-
7when
Syntax and Usage:
Master the concise syntax of the when expression in Kotlin.
Understand how to use when as a powerful replacement for switch statements in other languages.
Pattern Matching:
Explore the flexibility of when for pattern matching in Kotlin.
Learn to express complex conditions and match against various data types.
Multiple Conditions:
Discover how to handle multiple conditions within a single when expression.
Utilize the flexibility of when for clean and expressive decision-making in Kotlin code.
Smart Casting:
Leverage when for smart casting, allowing for concise and type-safe code.
Understand how when enhances code readability and reduces the need for explicit casting.
-
8Analyze Number
-
9Looping with for
Basic For Loop Syntax:
Understand the fundamental syntax of the for loop in Kotlin.
Iterating Over Ranges:
Learn how to use the for loop to iterate over ranges of numbers or other data types.
Collections Iteration:
Explore how the for loop simplifies iterating over collections like lists, arrays, or other iterable structures.
Looping with Indices:
Understand how to access both the elements and indices in a collection during iteration.
Advanced For Loop Techniques:
Dive into advanced techniques, including looping backwards, stepping, and using custom step values.
-
10Even Numbers
-
11Looping with while
Basic Syntax:
Understand the fundamental syntax of the while loop in Kotlin.
Loop Control:
Learn how to control the flow of execution using conditions within a while loop.
Increment and Decrement:
Explore techniques for incrementing or decrementing loop variables to control loop iterations.
Infinite Loops:
Recognize the concept of infinite loops and how to avoid unintended infinite execution.
Practical Examples:
Apply while loop constructs to solve real-world programming challenges
-
12Print Numbers Up To Ten
-
13do-while
Fundamentals of Do-While Loop:
Understand the syntax and usage of the do-while loop in Kotlin.
Explore how it differs from other loop constructs like while and for.
Iterative Execution:
Learn how the do-while loop executes statements repeatedly based on a specified condition.
Grasp the concept of "do first, check condition later."
Practical Applications:
Apply the do-while loop in solving real-world problems.
Explore scenarios where the do-while loop is particularly useful.
-
14CountDown From Ten
Functions and Lambdas
-
15Declaring classes and creating objects
Class Declaration:
How to define and structure classes in Kotlin.
Properties and Functions:
Declaring properties and member functions within a class.
Object Instantiation:
Creating instances (objects) of a class to represent real-world entities.
Constructors:
Understanding the role of constructors for initializing class properties during object creation.
Inheritance Basics:
Introduction to basic inheritance concepts for code reuse and extending class functionality.
-
16Display Car Info
-
17Constructors and initialization blocks
Creating Instances:
Understand how to create instances of classes using constructors.
Primary and Secondary Constructors:
Differentiate between primary and secondary constructors in Kotlin.
Initialization Blocks:
Explore initialization blocks for executing code during instance creation.
Default and Named Arguments:
Learn about default and named arguments for flexible constructor usage.
-
18Name Exercise
-
19Inheritance
Concept of Inheritance:
Understand the fundamental concept of inheritance in Kotlin.
Building Hierarchies:
Learn how to create class hierarchies and extend existing classes.
Code Reusability:
Explore how inheritance promotes code reusability and modularity.
Overriding Methods:
Master the technique of overriding methods to customize behavior in derived classes.
Superclass-Subclass Relationship:
Grasp the relationship between superclass and subclass and its significance in Kotlin.
-
20Polymorphism
Concept:
Understand the concept of polymorphism, a key object-oriented programming principle.
Implementation:
Learn how polymorphism is implemented in Kotlin through function overriding and interfaces.
Benefits:
Explore how polymorphism enhances code flexibility, extensibility, and maintainability.
Practical Application:
Apply polymorphism to create more adaptable and reusable code in Kotlin.
Examples:
Study real-world examples demonstrating polymorphic behavior in Kotlin applications.
-
21Vehicle Exercise
-
22Shape Exercise
-
23Interfaces
Interface Basics:
Understand the concept of interfaces as a way to define a contract for classes.
Implementing Interfaces:
Learn how classes in Kotlin implement interfaces to provide specific functionalities.
Default Implementations:
Explore the use of default implementations in interfaces for backward compatibility.
Multiple Interface Implementation:
Understand how Kotlin handles classes implementing multiple interfaces.
Interface Delegation:
Explore the concept of interface delegation as an alternative to traditional inheritance.
-
24Interface with Shapers
-
25Data Classes
Definition: Understand the concept and syntax of data classes in Kotlin.
Automatic Methods: Explore how data classes automatically generate essential methods like toString(), equals(), and hashCode().
Immutability: Learn how data classes contribute to creating immutable objects for concise and effective code.
Practical Use Cases: Apply data classes for streamlined representation of data in Kotlin, enhancing code readability and maintainability.
-
26Create Person with Data Classes
-
27Automatic generation of toString(), equals(), and hashCode()
toString():
Generate concise and readable string representations of Kotlin objects.
Simplify debugging and logging by automatically creating meaningful object representations.
equals():
Automatically generate consistent and accurate equality comparisons between objects.
Streamline the process of checking object equality without manual implementation.
hashCode():
Automate the creation of hash codes for objects, ensuring consistency with the equals() method.
Simplify the use of objects in hash-based collections.
-
28Person Creation with Automatic Generation
-
29Extension Functions
Extend existing Kotlin classes with new functionality.
Improve code readability by adding custom methods to standard types.
Enhance modularity and maintainability through the use of extension functions.
Gain a deeper understanding of Kotlin's expressive and versatile language features.
-
30String Extension Fun
Collections in Kotlin
-
31Declaring and invoking functions
Function Declaration:
How to declare functions in Kotlin, specifying parameters and return types.
Function Invocation:
The process of invoking or calling functions with appropriate arguments.
Default and Named Parameters:
How to define default parameter values and use named parameters for enhanced flexibility.
Function Expressions:
Writing concise function expressions and understanding their usage.
Lambda Expressions:
Introduction to lambda expressions as a powerful feature for defining anonymous functions.
-
32SumFunctionExercise
-
33Local functions
Definition and Scope:
Understand the concept of local functions within Kotlin.
Learn how local functions are scoped and how they can access variables in their surrounding functions.
Encapsulation and Code Organization:
Explore how local functions enhance encapsulation by keeping related code together.
Learn how to use local functions for better code organization and readability.
Benefits and Use Cases:
Recognize the advantages of using local functions in terms of code reuse and maintainability.
Discover practical use cases where local functions can contribute to more modular and efficient code.
Example Implementation:
Work through examples demonstrating the syntax and implementation of local functions in Kotlin.
Apply local functions in scenarios where their usage provides clear benefits.
-
34Sum and Square Calculation with Local Function
-
35Passing functions as parameters
Understand Higher-Order Functions:
Grasp the concept of higher-order functions in Kotlin.
Pass Functions as Parameters:
Learn the syntax and technique for passing functions as parameters to other functions.
Achieve Code Modularity:
Explore how passing functions enhances code modularity and promotes reusable and flexible code.
Apply in Practical Scenarios:
Apply the concept to real-world scenarios, gaining hands-on experience in utilizing functions as parameters.
-
36Function Operations: Higher-Order Functions
Exception Handling
-
37lists
Creating Lists:
Understand how to declare and initialize lists in Kotlin.
Common List Operations:
Explore basic operations like adding, removing, and updating elements in a list.
Iterating Through Lists:
Learn different ways to iterate through and access elements in a list.
List Manipulation Functions:
Explore Kotlin's built-in functions for sorting, filtering, and transforming lists.
Immutable and Mutable Lists:
Differentiate between immutable and mutable lists and their use cases.
-
38sets
No Duplicates:
Sets do not allow duplicate elements. If you try to add an element that already exists, it won't be added again.
Unordered:
Unlike lists, sets do not have a specific order for their elements. The order of elements is not guaranteed and may change.
Immutable and Mutable Sets:
Kotlin provides both immutable (Set) and mutable (MutableSet) sets. Immutable sets cannot be modified once created, while mutable sets can be updated.
Creation:
Sets can be created using the setOf() function for immutable sets and mutableSetOf() for mutable sets.
Common Operations:
Sets support common operations such as adding and removing elements, checking for the existence of an element, and performing set operations like union, intersection, and difference.
-
39maps
In the topic of "Maps," students will learn to efficiently manage and manipulate key-value pairs using Kotlin's Map data structure. They'll explore methods for insertion, retrieval, and iteration, gaining a strong understanding of how to leverage maps for effective data organization and retrieval in Kotlin programming.
-
40Functional operations on collections
Higher-Order Functions:
Understand the concept of higher-order functions and their role in functional programming.
Map, Filter, and Reduce:
Explore key functional operations like map for transformation, filter for selection, and reduce for aggregation on Kotlin collections.
Lambda Expressions:
Learn to use concise lambda expressions to pass functionality as parameters to these higher-order functions.
Chaining Operations:
Discover how to chain multiple functional operations together to create expressive and efficient transformations on collections.
-
41Using filter, map, and other collection functions
Filtering Data:
Master the filter function to selectively extract elements from collections based on specific criteria.
Transforming Data with Map:
Understand the map function to transform elements within a collection, creating new structures or modifying existing ones.
Chaining Operations:
Learn to chain multiple collection functions to perform complex transformations in a concise and expressive manner.
Exploring Additional Functions:
Explore other essential collection functions like flatMap, groupBy, and reduce to tackle diverse data manipulation scenarios.
Practical Application:
Apply these functions in real-world scenarios through hands-on exercises, reinforcing the ability to handle and process data effectively.
-
42Chaining operations
Chaining Operations:
Understand the concept of chaining operations in Kotlin.
Learn how to efficiently link and execute multiple operations in a concise and readable manner.
Explore practical examples and scenarios where chaining enhances code readability and maintainability.
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don't have an internet connection, some instructors also let their students download course lectures. That's up to the instructor though, so make sure you get on their good side!
Please, login to leave a review