Currently Empty: £0.00
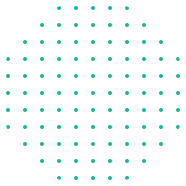
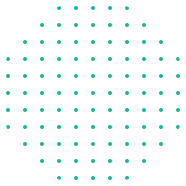
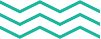
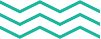
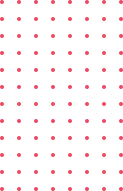
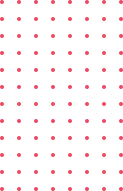
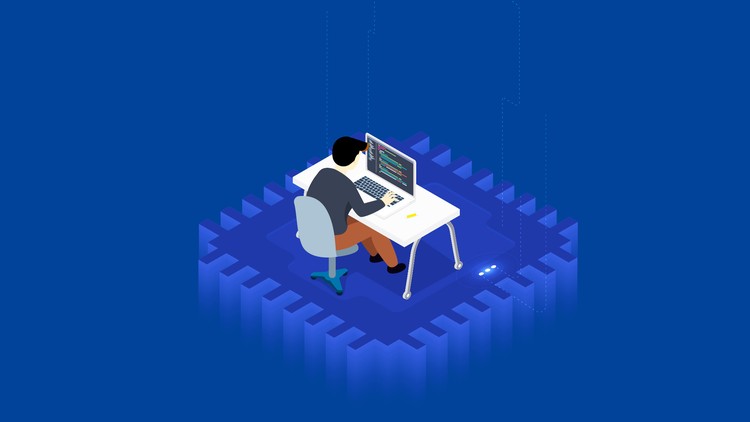
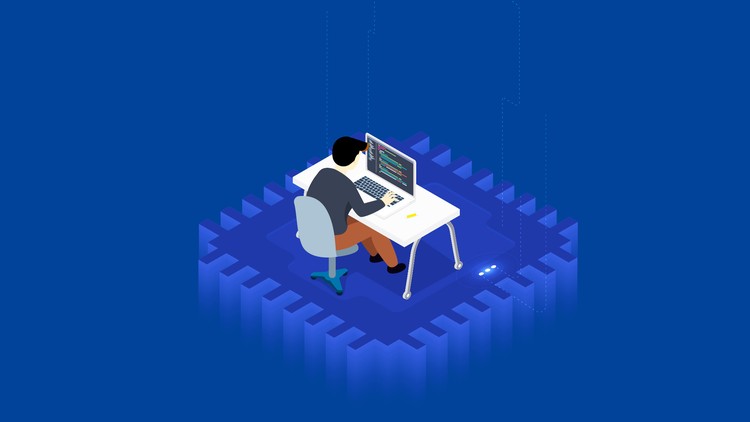
In this course we’ll understand what can cause performance issues in our applications, and how to resolve them. This includes a review of some of the options available to us as developers at design-time – how to make good coding choices for optimal performance. For example, when should you pick an ArrayList over a LinkedList? How much difference does the StringBuilder really make? Is Lambda syntax more or less efficient at certain operations? We’ll also learn about various ways that we can configure the virtual machine to provide better performance at run-time, with a range of runtime arguments. We’ll also be diving deep into how the virtual machine manages memory, and how the garbage collection process works and impacts on application performance.
Along the way we’ll be learning about the Just In Time compiler, performance testing and benchmarking, decompiling bytecode, using the GraalVM as an alternative virtual machine and more.
About Java Versions: This is the second iteration of this course and it is designed for all the current versions of Java that have long term support (Java 8 , Java 11 and Java 17). You can follow along with any of these versions. You can also use either the Oracle or the OpenJDK JVMs. For developers using other JVM languages (such as Kotlin, Scala and Groovy) all of the JVM configuration parts of this course will still be relevant, and some of the coding choices may be useful to consider also. (Note that there’s even a review of whether Kotlin provides better or worse performance than Java!)
Chapter 2 - Just In Time Compilation and the Code Cache
Chapter 3 - Selecting the JVM
-
6What is bytecode?
-
7The concept of "Just In Time Compilation"
-
8Introducing the first example project
-
9Finding out which methods are being compiled in our applications
-
10The C1 and C2 Compilers and logging the compilation activity
-
11Tuning the code cache size
-
12Remotely monitoring the code cache with JConsole
Chapter 4 - How memory works - the stack and the heap
Chapter 5 - Passing objects between methods
Chapter 6 - Memory exercise 1
Chapter 7 - Escaping References
Chapter 8 - Memory Exercise 2
-
28Introduction - what is an escaping reference?
-
29Strategy 1 - using an iterator
-
30Strategy 2 - duplicating collections
-
31Strategy 3 - using immutable collections
-
32Strategy 4 - duplicating objects
-
33Strategy 5 - using interfaces to create immutable objects
-
34Strategy 6 - using modules to hide the implementation
Chapter 9 - The Metaspace and internal JVM memory optimisations
Chapter 10 - Tuning the JVM's Memory Settings
Chapter 11 - Introducing Garbage Collection
Chapter 12 - Monitoring the Heap
-
47What it means when we say Java is a managed language
-
48How Java knows which objects can be removed from the Heap
-
49The System.gc() method
-
50Java 11's garbage collector can give unused memory back to the operating system
-
51Why it's not a good idea to run the System.gc() method
-
52The finalize() method
-
53The danger of using finalize()
Chapter 13 - Analysing a heap dump
Chapter 14 - Generational Garbage Collection
Chapter 15 - Garbage Collector tuning & selection
Chapter 16 - Using a profiler to analyse application performance
-
65Monitoring garbage collections
-
66Turning off automated heap allocation sizing
-
67Tuning garbage collection - old and young allocation
-
68Tuning garbage collection - survivor space allocation
-
69Tuning garbage collection - generations needed to become old
-
70Selecting a garbage collector
-
71The G1 garbage collector
-
72Tuning the G1 garbage collector
-
73String de-duplication
Chapter 17 - Assessing Performance
-
74Introducing Java Mission Control (JMC)
-
75Building the JMC binaries
-
76Running JMC and connecting to a VM
-
77Customising the overview tab
-
78The MBean Browser tab
-
79The System, Memory and Diagnostic Commands tabs
-
80Introducing our problem project
-
81Using the flight recorder
-
82Analyzing a flight recording
-
83Improving our application
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don't have an internet connection, some instructors also let their students download course lectures. That's up to the instructor though, so make sure you get on their good side!
Stars 5
2528
Stars 4
1457
Stars 3
278
Stars 2
39
Stars 1
26