Currently Empty: £0.00
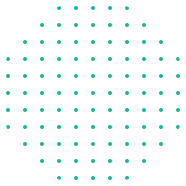
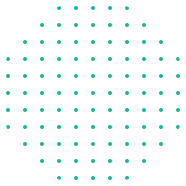
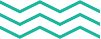
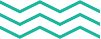
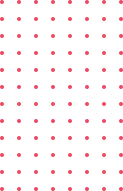
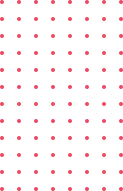
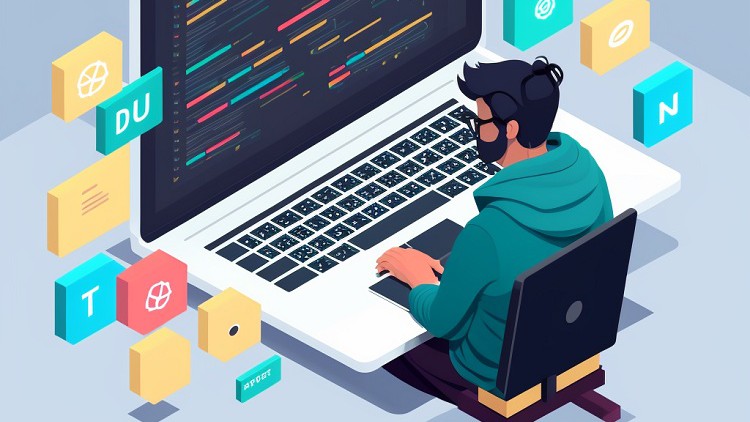
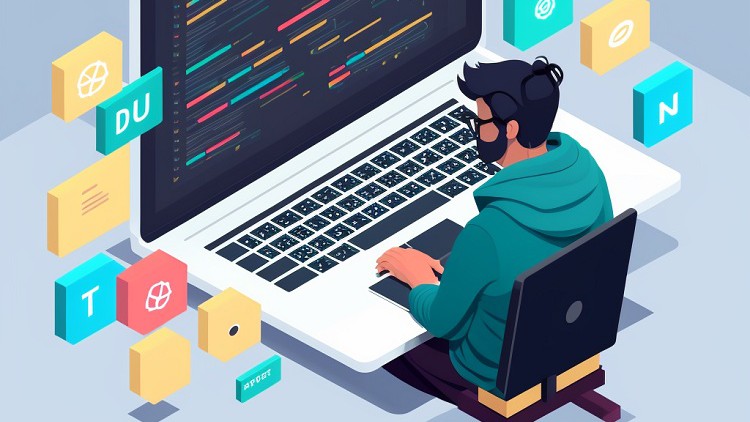
In this course, you will learn how to use Micronaut and Kotlin to create fast, lightweight and reactive web applications.
Micronaut is a modern framework for building microservices and serverless applications with Kotlin. It offers compile-time dependency injection, AOP, configuration management, HTTP client and server, and GraalVM support.
Kotlin is a concise and expressive language that runs on the JVM and can interoperate with Java. It offers many features that make web development easier and more enjoyable, such as null safety, data classes, coroutines, extension functions, and DSLs.
You will explore the main features and benefits of Micronaut and Kotlin through a series of hands-on exercises. You will learn how to create REST APIs with Micronaut and test your applications with Micronaut Test and JUnit 5.
You will also learn how to use Micronaut Data to interact with relational (E.g. MySQL/Postgres) and non-relational (E.g., MongoDB) databases.
This course is suitable for developers who have some basic knowledge of Kotlin and web development, as well as those who want to explore new frameworks and tools. By the end of this course, you will have a solid understanding of how to use Micronaut and Kotlin to create scalable and resilient web applications.
Introduction to Micronaut Controllers
-
1Introduction
In this section, you will get an overview of Micronaut, a modern framework for building microservices and serverless applications with Kotlin.
You will learn about the main features and benefits of Micronaut, such as dependency injection, configuration management, HTTP client and server, etc. You will also learn about some of the popular tools and technologies we will work with in this course such as Kafka, MongoDB, JOOQ, Micronaut Data JDBC, and Redis
-
2Creating a Micronaut Project
In this lecture, you will learn how to create and configure a simple Micronaut project from scratch.
Micronaut Configuration
Data Persistence and Controller Basic
Controllers
-
6Entities and Data Transfer Objects
In this lesson, you will create a simple product entity class that will represent data in your database.
-
7Repositories Save - Memory database
In this lesson, you will learn how to create a simple repository for a database table. We will start to implement common functions for creating, reading, updating and deleting data from the database.
-
8Repository Test
You will learn how to write simple tests for the save function.
-
9Repository List - Memory database
In this lecture, you will start implementing a list function for your repository. You will learn how to write an appropriate test for your list function.
-
10Repository Update - Memory database
In this lecture, you will learn how to implement an update function, and write a test using Micronaut Test and Junit5
-
11Repository Delete - Memory database
In this lecture, you will write a test for a delete function with Micronaut Data JDBC, and then make the test pass, by implementing a working delete function.
Flyway Setup
-
12Controller GET
In this lecture, you will learn how to implement a working controller GET endpoint. You will learn about some possible causes for common errors you could encounter during implementation. You will also learn how to write appropriate tests for the endpoint.
-
13Controller Save
In this lecture, you will learn how to create a test for a POST endpoint using Micronaut Test, Micronaut Http client and Junit.
-
14Controller POST implementation
In this lesson, you will learn how to implement a working POST endpoint and make your test to pass.
-
15Controller PUT Test
In this lecture, you will learn how to create a test for a PUT endpoint using Micronaut Test, Micronaut Http client and Junit.
-
16Controller PUT Implementation
In this lecture, you will learn how to implement a working PUT endpoint. Your endpoint implementation will make your test to pass, and it will successfully update database records as specified.
-
17Controller DELETE Test
In this lecture, you will learn how to create a test for a DELETE endpoint using Micronaut Test, Micronaut Http client and Junit.
-
18Controller DELETE Implemenation
In this lecture, you will learn how to implement a DELETE endpoint, and make the test to pass.
-
19Controller GET (List) Test
In this lecture, you will learn how to create a test for a GET endpoint that returns a list of items. You will make use of Micronaut Http client and Junit.
-
20Controller GET (List) Implementation
In this lecture, you will learn how to implement a GET endpoint that returns a list of items, and make the test written in our previous lecture to pass.
Micronaut Data JDBC
JOOQ integration
-
22Database access with JDBC
In this lecture, we will implement a simple product repository using Micronaut Data JDBC. Your repository will have basic functionality like CREATE, READ, UPDATE, and DELETE for interacting with your underlying database management system.
-
23Serving DB data via controller/jdbc
In this lecture, you will learn how to implement a simple endpoint that retrieves data from the database and serves it to clients via the endpoint.
Redis Integration
-
24Setup and configure Jooq
In this lecture, you will learn how to install and configure JOOQ for your Micronaut Project.
-
25Configuring Jooq db connection
In this lecture, you will learn about Providers and how to create a DSLContext provider to allow JOOQ to connect to the database and execute queries.
-
26Repository - save, findbyId
In this lecture, we will create a simple repository class that makes use of JOOQ.
-
27Repository List with JOOQ
In this lecture, you will learn how to implement a list function with Micronaut JOOQ, and write a test using Micronaut Test and Junit5
-
28Jooq repository update
In this lecture, you will learn how to implement an update function with Micronaut JOOQ, and write a test using Micronaut Test and Junit5
-
29JOOQ repository delete
In this lecture, you will learn how to implement a delete function with Micronaut JOOQ, and write a test using Micronaut Test and Junit5
-
30Jooq repository delete all
In this lecture, you will learn how to implement a deleteAll function with Micronaut JOOQ, and write a test using Micronaut Test and Junit5
-
31Controller get with jooq repository
Kafka Integration
-
32Redis setup and configuration
In this lecture, you will learn how to integrate Redis and configure it for use with Micronaut.
-
33Redis Caching
In this lecture, you will learn how to implement caching with Redis for a simple endpoint. You will also learn how to test your implementation using Micronaut Test and Junit5.
Mongo Integration
-
34Kafka Setup and Configuration
In this lecture, you will learn how to setup and configure Kafka for your project.
-
35Kafka Client
In this lecture, you will learn how to create a simple Kafka Client that can produce messages to a Kafka Topic.
-
36Kafka Listener
In this lecture, you will learn how to create a Kafka Listener, that can read messages from Kafka topics.
-
37Kafka Integration testing
In this lesson, you will learn how to test your Kafka setup, including your listeners and clients.
-
38Kafka Processing
In this lesson, you will learn how to implement a simple kafka processor. You will read messages from a Kafka Topic and persist the data into a database.
-
39Kafka Processing Test
In this lecture, you will learn how to write integration tests for your Kafka processors.
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don't have an internet connection, some instructors also let their students download course lectures. That's up to the instructor though, so make sure you get on their good side!
Stars 5
3
Stars 4
1
Stars 3
2
Stars 2
1
Stars 1
3