Currently Empty: £0.00
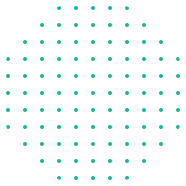
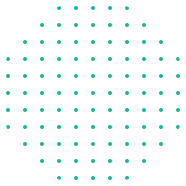
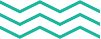
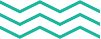
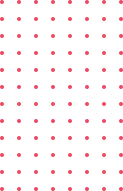
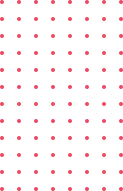
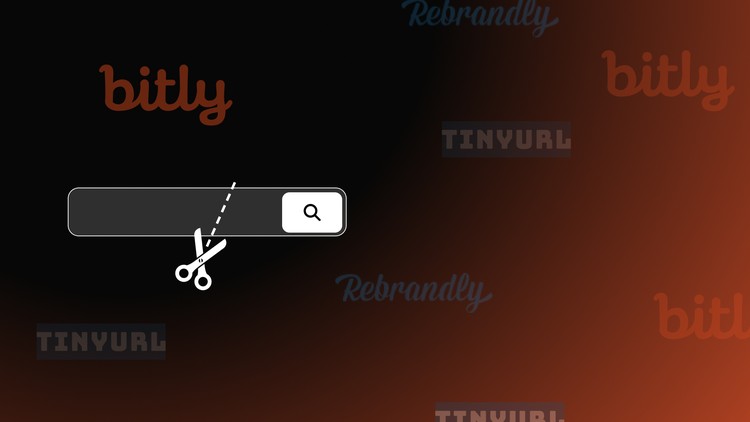
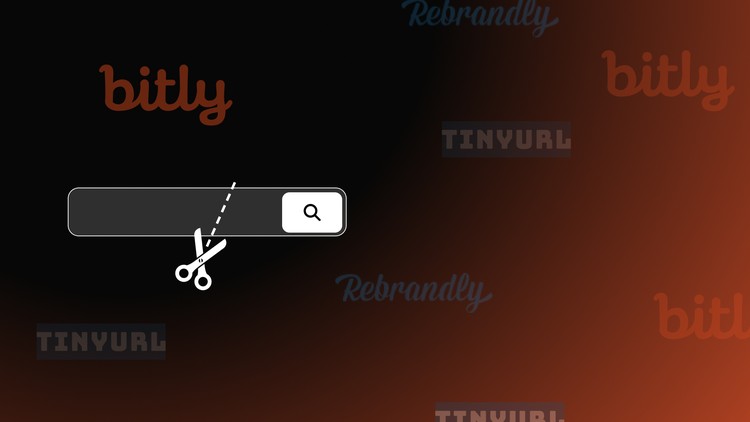
Want to learn how to build awesome web application with ASPNET? This course is for you! We’ll show you how to create your own cool URL shortener using ASP.NET Core. You’ll build the whole thing – the part users see (front-end) and the behind-the-scenes magic (back-end). Plus, you’ll learn how to store info safely in a database with Entity Framework Core.
What will you learn?
-
Make a user-friendly website: Build the part of your URL shortener where people log in and manage their links (using ASP.NET MVC). Think of it like the control panel for your app.
-
Master those redirects: Set up a special system (ASP.NET Web API) to make sure shortened links take people to the right place. It’s like the super-fast traffic cop for your website!
-
Get organized with a database: Use Entity Framework Core to store and manage your shortened links. Imagine a super neat filing cabinet for all your website’s important info.
-
Become a full-stack pro: Learn how to fit all the pieces together (ASP.NET MVC, Web API, and Entity Framework Core) to create a complete web app.
This course is super hands-on, so you’ll finish feeling like you can build your own amazing websites with .NET Core. Ready to get started? Let’s do this!
Intro to the course
Introduction to ASP.NET MVC
-
2What to expect from this course?
What will you learn in this course? ?
- ? Make a simple web link shortener.
- ?️ Three projects: Admin site, Link Redirector, and Data Management.
- ?️ Start with basic SQL database, move to Azure SQL.
- ? Learn to use Entity Framework for handling data.
- ? Manage your code with GitHub.
- ? Keep your app safe with ASP.NET Identity.
- ? Use Bootstrap for easy and good-looking design.
- ☁️ Put your app online with Azure Web Services.
-
3What you should know?
-
4Setting up development environment
To get the most out of this course, I recommend that you follow along while I code. However, for you to be able to do so, there are some tools that you need to install.
-
5Github Branching and Pushing Your Code to Github Repository
To manage your code, you will use a GitHub repository. In this section, you will learn how I will structure my code throughout this course and how to push my code to a new GitHub repository.
-
6Quiz: Intro to the course
Passing Data From Controller to View in ASP.NET MVC
-
7What is ASP.NET MVC?
ASP.NET MVC is a web application framework developed by Microsoft, which implements the model-view-controller (MVC) design pattern. It enables developers to build scalable and maintainable web applications by separating the application's concerns into three main components: the Model (data), the View (UI), and the Controller (business logic).
-
8ASP.NET MVC Project Default Files
ASP.NET MVC comes with a set of default files that form the backbone of your web application. To understand these files and how they work together, check out this video. It's a great resource for getting started with ASP.NET MVC and lays a solid foundation for your development journey.
-
9Creating Your First Controller in ASP.NET MVC
Creating your first controller is a pivotal step in ASP.NET MVC development. This video will guide you through the process, showing you how to set up, structure, and implement your first controller effectively. It's an essential watch for beginners looking to master the fundamentals of ASP.NET MVC controllers.
-
10Creating Your First Model in ASP.NET MVC
Creating your first Model in ASP.NET MVC is an essential step in defining the data structure of your application. This video tutorial will guide you through the process of creating and utilizing your first Model, teaching you how to define properties. It's crucial for effectively managing data and ensuring seamless interaction between your database and user interface in ASP.NET MVC applications.
-
11Creating Your First View in ASP.NET MVC
Creating your first View in ASP.NET MVC is a key step in building your web application's user interface. This video will walk you through the process of creating your first View. You'll learn how to integrate C# code in HTML using Razor syntax.
-
12Quiz: Introduction to ASP.NET MVC
Introduction to Application Controllers, Models and Views
-
13Introduction to Passing Data From Controller to a View
Discover the key methods for passing data from a controller to a view in MVC in this concise video. I'll be highlighting the different approaches, including ViewData, ViewBag, TempData, and Model Binding, explaining their unique characteristics and differences.
-
14Using a Model to Pass Data from a Controller to a View
In ASP.NET MVC, models are used to pass data from the controller to the view. A model typically is a class that represents the data of the application. When a controller handles a request, it can create and populate a model with data retrieved from a database or another source. This model is then passed to the view, which uses it to render the appropriate HTML to the user.
This pattern helps in separating the concerns of data management (model), request handling (controller), and user interface (view), making the code more organized and maintainable.
-
15Using ViewData to Pass Data from a Controller to a View
In ASP.NET MVC, ViewData is a dictionary object used to pass data from a controller to a view. It's a dynamic property of the ControllerBase class, allowing you to add any object with a key-value pair. Since it's loosely typed, you don't need to define a specific class for the data you're passing.
However, this lack of type safety requires casting in the view and can lead to runtime errors if not used carefully. ViewData is useful for passing small amounts of data, like dropdown lists or configuration settings, but for more complex data structures, using a model is recommended.
-
16Using ViewBag to Pass Data from a Controller to a View
In ASP.NET MVC, ViewBag is a dynamic property provided by the Controller base class used for passing data from the controller to the view. It allows you to add properties to the `ViewBag` and then access them in the view with the same names. Like `ViewData`, it is dynamic and doesn't require pre-defined classes, offering flexibility but lacking type safety. This means there's no compile-time checking, which can lead to runtime errors if properties are not used correctly. `ViewBag` is ideal for transferring small pieces of data, such as titles, labels, or dropdown list items, from the controller to the view.
-
17Using TempData to Pass data from a Controller to a View
In ASP.NET MVC, TempData is used to pass data from the controller to the view, but it's designed for temporary storage, meaning it's meant to be used for a single redirect. TempData is a dictionary stored in session state and is capable of keeping data for the duration of an HTTP request. It's ideal for scenarios such as passing error messages or status data after a redirect, where ViewData and ViewBag would not persist the data after the redirect. Once TempData is read in the view, the information is discarded.
-
18ASP.NET MVC Data Passing Methods Quiz
Test your knowledge on various methods of passing data from controllers to views in ASP.NET MVC. This quiz covers key concepts such as ViewData, ViewBag, TempData, and strongly-typed models, ensuring you understand their usage and differences.
Methods for Passing Data from a View to a Controller
-
19Introduction to Application Controllers, Models and Views
On the next parts you will start building the app step by step. You will create the key Controllers, Models and Views.
-
20Cleaning up the Homepage Controller
In this part you will clean up the HomeController by removing unused actions and Views to enhance performance and maintainability.
-
21Designing the Homepage
You will learn how to design a homepage using custom CSS for unique styles and Bootstrap for responsive, pre-designed components, achieving both personalized aesthetics and functionality.
-
22Designing the Navigation Bar
On this part you will learn how to design the navigation bar and update the content of the footer.
-
23Designing the All Links Page
The "All Links" page is where each user will see their shortened links, and the Administrator will see all links from all users. On this part, you will learn how to design it using the Bootstrap table component.
-
24Passing Fake Data to All Links Page
On this part you will learn how to pass dummy data from the Controller to the All Links page. You will pass the data using a model.
-
25What is a ViewModel in ASP.NET MVC
ASP.NET MVC ViewModel is used to organize and pass data from the controller to the view, making it easier to display specific data on web pages. It helps keep code clean and maintainable.
-
26Creating the User Model
This is the first part of creating the User section where as an Administrator you can check all the users of the application and also the links that each user has created.
-
27Creating User Controller and View
-
28Updating Footer Style
-
29Designing the Login Form
-
30Designing the Register Form
-
31Application Controllers, Models and Views Quiz
Test your knowledge on the essential concepts of ASP.NET MVC, including controllers, models, views, and data passing methods. This quiz covers topics such as designing user interfaces, implementing user models, and best practices for creating dynamic web applications. Perfect for developers looking to reinforce their understanding of ASP.NET MVC framework and improve their web development skills.
Data Validation and Form Submission
-
32Introduction
-
33Passing Data in Query Strings
-
34Passing Parameters as Route Data
-
35Passing Data with Form Submission
-
36Form Data Submission view ViewModel
-
37Passing Data to Controller with Ajax
-
38Passing Data from a View to a Controller
Assess your understanding of various methods for passing data from a view to a controller in ASP.NET MVC. This quiz covers key concepts including query strings, route data, form submissions, ViewModels, and Ajax, providing a comprehensive review of effective data transfer techniques in web development.
Setting up Entity Framework Core in ASP.NET
-
39Introduction
What to expect from this chapter?
-
40Passing URL Value to HomeController
-
41URL Format Validation with RegEx
Learn how to ensure URLs are correctly formatted using Regular Expressions (RegEx) in your applications. This video guides you through creating and implementing RegEx patterns to validate URLs, enhancing data integrity and user input validation in your software projects.
-
42Handling Login Form Submission
This video tutorial covers the essentials of handling login form submissions in ASP.NET MVC.
-
43Login Form Data Validation with Data Annotations
Discover how to implement data validation in your .NET applications using annotations. This guide explores the power of data annotations to simplify validation logic, enforce business rules, and ensure data integrity by annotating model properties directly in your code. Perfect for developers looking to streamline their validation processes.
-
44Handling Register Form Submission
This video tutorial covers the essentials of handling register form submissions in ASP.NET MVC.
-
45Register Form Data Validation
Learn how to validate data of a register button like, fullname, email, and password strength. Here you will also learn how to validate if the Password and Confirm Password match.
-
46Creating a Custom Validation Attribute (Email validator)
Creating a custom validation attribute for email validation in .NET involves defining a class that inherits from the ValidationAttribute class. This custom attribute can then be used to annotate model properties to ensure they hold valid email addresses.
-
47Data Validation and Form Submission
Evaluate your understanding of data validation and form submission techniques in ASP.NET MVC. This quiz covers essential topics such as URL value passing, regular expression validation, handling login and registration forms, and implementing custom validation attributes. Ideal for developers aiming to strengthen their skills in ensuring data integrity and security in web applications.
Manage Data Using Entity Framework
-
48What You Will Learn?
Entity Framework is an ORM that simplifies data access in .NET applications by letting you interact with databases using C# code. It offers benefits like increased productivity, strongly typed code, and a code-first approach.
-
49Installing Entity Framework Packages
In this quick tutorial, you'll learn how to install the powerful Entity Framework (EF) packages using NuGet. We'll cover finding the right package and adding it to your project in a few simple steps.
-
50Creating the Application Models
In the ASP.NET MVC framework, a model plays a crucial role in representing and managing the core data of your application
-
51Creating the DbContext File of Entity Framework
In Entity Framework (EF), the DbContext file is the heart of your interaction with the database. It acts as a bridge between your object-oriented code and your relational data.
Your DbContext class defines DbSet properties that correspond to tables in your database. Each DbSet represents a collection of entities of a specific type.
The DbContext provides methods for querying your database (using LINQ), saving changes to entities, and managing the database connection. -
52Configuring the AppDbContext File
Configuring the AppDbContext file is crucial because it's the blueprint your application uses to interact with your database. It defines the shape of your data, the database connection details, and any special rules your application needs to follow when working with the data. A well-configured AppDbContext ensures smooth communication between your app and its data store.
-
53Adding Database Migrations
Adding migrations in database development allows you to track and manage changes to your database schema in a structured way. These migrations hold instructions to modify the database, enabling you to easily apply updates or revert to earlier versions if needed.
-
54Modifying Database Schema with Migrations
Migrations offer a controlled and systematic way to modify the structure of your database schema. By creating migration files, you define precise changes such as adding or removing tables, altering columns, or adjusting data types, ensuring that your database evolves alongside your application's requirements.
-
55Creating a Database Seeder File
Database seeder files provide a convenient way to populate your database with sample data. This is particularly useful for testing, demonstrations, or setting up a fresh development environment. Within the seeder file, you define the data you want to insert into specific database tables.
-
56Setting up Entity Framework Core in ASP.NET Quiz
Test your understanding of setting up and configuring Entity Framework Core in ASP.NET. This quiz covers key concepts including installing packages, creating models, configuring the DbContext, and managing database migrations and seed data.
Building and Configuring ASP.NET Core MVC Services
-
57Getting Data From DataBase with Entity Framework
In this tutorial, you will learn how to use Entity Framework to retrieve data from your database. You'll start by learning about dependency injection, how to inject your DbContext into your controller, and then use it to fetch the data.
-
58Shorten a URL and Store It in Database with Entity Framework
In this tutorial, you will learn how to shorten a URL and store it in the database with Entity Framework. To shorten a URL you will create a custom function which is going to generate a random string.
-
59Using TempData to Show a Success Notification
In this tutorial, you'll learn how to use TempData to pass information between actions, enabling you to display a notification message on the home page after a URL is shortened successfully.
-
60Removing Data from Database with Entity Framework
In this tutorial, you will learn how to remove data from database with Entity Framework and also redirect user to the same page after the data is removed.
-
61Loading Users From Database with Entity Framework
In this tutorial, you will learn how to load users from the database using Entity Framework.
-
62Improving Users View with More Information
In this tutorial, you will learn how to improve the user's view with more information. You will first add the total number of links and clicks, as well as a nested section where you can show the list of all the links with their click numbers.
-
63Improving All Links View with User Information
In this tutorial, you will learn how to improve the All Links view with more information. For that you will first create a navigational property for the user, create a view model for the user, reference it and also update the select statement.
-
64Manage Data Using Entity Framework Quiz
Evaluate your understanding of managing data using Entity Framework in ASP.NET. This quiz covers essential operations such as retrieving, adding, updating, and deleting data, as well as enhancing views with user information and notifications.
Asynchronous Programming in ASP.NET MVC
-
65Why Use Services in ASP.NET?
In this part, you will learn why should you use services in an ASP.NET application?
-
66Why Use Service Interfaces in ASP.NET?
In this part, you will learn why you need to create and use interfaces with your services in an ASP.NET application.
-
67Defining IUrlsService Interface
On this part you will define the IUrlsService Interface method signatures it will contain. You will define the return types, method names and parameters
-
68Implement Users Service Methods
In this part, you will implement all the methods of the IUsersService interface. This will cover adding new users to the database, removing users, and updating existing user information.
-
69Implement Urls Service Methods
In this part, you will implement all the methods of the IUrlsService interface. This will cover adding new urls to the database, removing urls, and updating existing url information.
-
70Update UrlsController to Use UrlsService instead of DbContext
In this part, you will learn how to inject the IUrlsService interface into the UrlsController and use its methods to retrieve, send, and update data in the database.
-
71Choosing the Right Service Lifetime (AddTransient, AddScoped, AddSingleton)
In ASP.NET Core, AddTransient, AddScoped, and AddSingleton dictate how long instances of your services persist within the application. AddTransient creates a new instance each time, AddScoped provides a single instance per request, and AddSingleton offers a single instance throughout the application's lifetime.
-
72Update AuthenticationController to Use IUsersService
In this part, you will replace the DbContext injection in the AuthenticationController with IUsersService. You will use this service to perform all the operations.
-
73Adding Automapper to Your ASP.NET Applications
AutoMapper simplifies object-to-object mapping in your ASP.NET Core applications. This eliminates tedious manual mapping code, improving code readability and maintainability.
-
74Building and Configuring ASP.NET Core MVC Services Quiz
Test your understanding of building and configuring ASP.NET Core MVC services. This quiz covers key concepts such as the use of services, service interfaces, implementing service methods, choosing service lifetimes, and integrating libraries like AutoMapper.
Securing Your Applications with Authentication and Authorization
-
75Introduction to Asynchronous Programming
-
76Converting UsersService from Synchronous to Asynchronous
In this part, you will learn how to convert the UsersService classes synchronous methods to asynchronous methods.
-
77Converting UsersController from Synchronous to Asynchronous
In this part, you will learn how to convert the UsersController from synchronous to asynchronous controller.
-
78Converting UrlsService from Synchronous to Asynchronous
In this part, you will learn how to convert the UrlsService classes synchronous methods to asynchronous methods.
-
79Converting UrlsController from Synchronous to Asynchronous
In this part, you will learn how to convert the UrlsController from synchronous to asynchronous controller.
-
80Quiz: Asynchronous Programming in ASP.NET MVC
Assess your knowledge of asynchronous programming in ASP.NET MVC. This quiz covers fundamental concepts, as well as the process of converting synchronous services and controllers to asynchronous ones, ensuring efficient and responsive web applications.
How long do I have access to the course materials?
You can view and review the lecture materials indefinitely, like an on-demand channel.
Can I take my courses with me wherever I go?
Definitely! If you have an internet connection, courses on Udemy are available on any device at any time. If you don't have an internet connection, some instructors also let their students download course lectures. That's up to the instructor though, so make sure you get on their good side!
Stars 5
15
Stars 4
6
Stars 3
2
Stars 2
1
Stars 1
0